一、控件和布局
1. QGroupBox
QGroupBox为构建分组框提供了支持
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35 | MainWindow::MainWindow(QWidget *parent) : QWidget(parent) {
QVBoxLayout *layout = new QVBoxLayout;
//创建两组
QGroupBox *box1 = new QGroupBox;
QVBoxLayout *layout1 = new QVBoxLayout;
box1->setLayout(layout1);
QGroupBox *box2 = new QGroupBox;
QVBoxLayout *layout2 = new QVBoxLayout;
box2->setLayout(layout2);
QRadioButton *btn1 = new QRadioButton("抽烟");
QRadioButton *btn2 = new QRadioButton("喝酒");
QRadioButton *btn3 = new QRadioButton("烫头");
QRadioButton *btn4 = new QRadioButton("男");
QRadioButton *btn5 = new QRadioButton("女");
QRadioButton *btn6 = new QRadioButton("妖");
layout1->addWidget(btn1);
layout1->addWidget(btn2);
layout1->addWidget(btn3);
layout2->addWidget(btn4);
layout2->addWidget(btn5);
layout2->addWidget(btn6);
//把两组放到整体布局中
layout->addWidget(box1);
layout->addWidget(box2);
setLayout(layout);
}
|
运行程序:
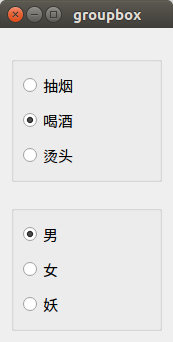
工具箱是一个窗口小部件,它一个接一个地显示一列选项卡
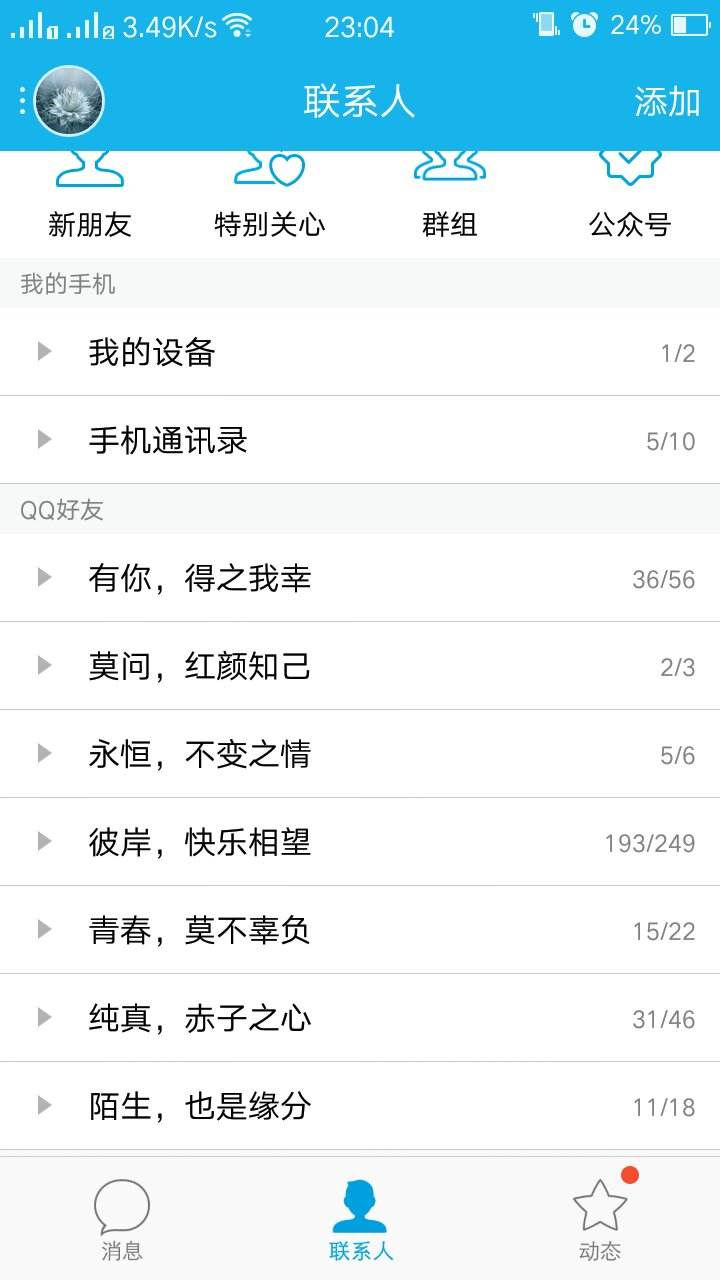
通过QToolBox可以实现QQ中的选项卡功能
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34 | MainWindow::MainWindow(QWidget *parent) : QWidget(parent) {
QVBoxLayout*layout = new QVBoxLayout;
QToolBox*box = new QToolBox;
//条目
QGroupBox *gb1 = new QGroupBox;
QVBoxLayout *layout1 = new QVBoxLayout;
gb1->setLayout(layout1);
QLabel *label1 = new QLabel("张三");
QLabel *label2 = new QLabel("李四");
QLabel *label3 = new QLabel("王五");
layout1->addWidget(label1);
layout1->addWidget(label2);
layout1->addWidget(label3);
//条目
QGroupBox *gb2 = new QGroupBox;
QVBoxLayout *layout2 = new QVBoxLayout;
gb2->setLayout(layout2);
QLabel *label4 = new QLabel("周期");
QLabel *label5 = new QLabel("粥吧");
QLabel *label6 = new QLabel("啁啾");
layout2->addWidget(label4);
layout2->addWidget(label5);
layout2->addWidget(label6);
//添加选项卡
box->addItem(gb1,"初中同学");
box->addItem(gb2,"高中同学");
layout->addWidget(box);
setLayout(layout);
}
|
运行程序:

QTableWidget是QT程序中常用的显示数据表格的空间
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18 | MainWindow::MainWindow(QWidget* parent):QWidget(parent) {
// setFixedSize(1000,1000);
//设置行和列数
QTableWidget *w = new QTableWidget(4,4,this);
w->setFixedSize(600,600);
//标题列表
QStringList l{"姓名","年纪","成绩","性别"};
//设置标题
w->setHorizontalHeaderLabels(l);
//创建条目
QTableWidgetItem *item1 = new QTableWidgetItem("张三");
QTableWidgetItem *item2 = new QTableWidgetItem("30");
//添加条目
w->setItem(0,0,item1);
w->setItem(0,1,item2);
}
|
setHorizontalHeaderLabels:设置标题栏
setItem:添加条目
运行程序:
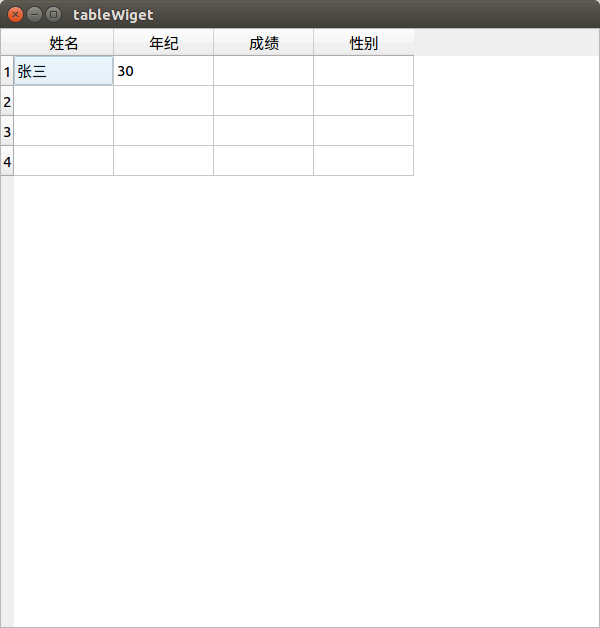
4. QGridLayout
格栅布局,也被称作网格布局(多行多列)。栅格布局将位于其中的窗口部件放入一个网状的栅格之中
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26 | MainWindow::MainWindow(QWidget* parent):QWidget(parent) {
const char *buttontext[20] =
{
"7", "8", "9", "+", "(",
"4", "5", "6", "-", ")",
"1", "2", "3", "*", "<-",
"0", ".", "=", "/", "C"
};
QVBoxLayout *layout = new QVBoxLayout;
//输入框
QLineEdit *edit = new QLineEdit;
layout->addWidget(edit);
//网格布局
QGridLayout *layout1 = new QGridLayout;
for (int i = 0; i < sizeof(buttontext) / sizeof(buttontext[0]); ++i) {
QPushButton *btn = new QPushButton(buttontext[i]);
layout1->addWidget(btn,i/5,i%5);
}
layout->addLayout(layout1);
//设置布局
setLayout(layout);
}
|
运行程序:
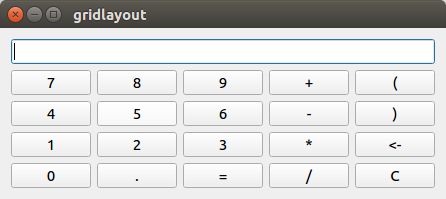